Realizar un programa que le permita al usuario ingresar una serie de datos de un libro como el nombre del autor, el titulo, el precio y código.
El programa deberá mostrar un menú que nos pida insertar registro, mostrar registro y buscar un registro.
Todos los datos deberán ser almacenados en un archivo .txt en forma de bits.
Captura de muestra:
Clase Principal DLibros:
package dlibros; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import java.awt.*; import javax.swing.*; public class DLibros { // Objeto agenda static Datos[]obj=new Datos[3]; //Menu para elegir una opcion public static int menu(){ String opt=JOptionPane.showInputDialog(null,"" + "<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:insert1.png'/>1. Insertar registro" + "n<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:list1.png'/>2. Mostrar registro" + "n<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:search1.png'/>3. Buscar un registro" + "n<html><p style="width: 500px; background-color: #CCCCCC; border-left: solid 20px #FF0000; padding: 10px; font-family: consolas; font-size: 30px;"><b><img src='file:leave1.png'/>4. Salirn","ELIGE UNA OPCION",JOptionPane.PLAIN_MESSAGE); int op=Integer.parseInt(opt); return op; } //Main principal public static void main(String[] args) throws FileNotFoundException, IOException,ClassNotFoundException{ int x=0; do{ x=menu(); switch(x){ case 1: //Captura datos try{ for(int i=0;i<3;i++){ obj[i]=new Datos(); obj[i].captura(); } FileOutputStream a=new FileOutputStream("Registro.txt"); ObjectOutputStream aa=new ObjectOutputStream(a); aa.writeObject(obj); aa.flush(); aa.close(); } catch(IOException e){ Icon icono = new ImageIcon("C:\Users\Ortuño\Documents\NetBeansProjects\Dialogos\error.png"); JOptionPane.showMessageDialog(null,"Error al escribir !","MENSAJE",JOptionPane.ERROR_MESSAGE,icono); } break; case 2: // Abrir el archivo (Imprimir) FileInputStream b=new FileInputStream("Registro.txt"); ObjectInputStream bb=new ObjectInputStream(b); obj=(Datos[])bb.readObject(); bb.close(); JTextArea Salida1= new JTextArea(); String cad1="LISTA:n"; for(int i=0;i<3;i++){ cad1+=("n"+obj[i].autor+" "+obj[i].titulo+" "+obj[i].precio+" "+obj[i].codigo+"n"); Salida1.setText(cad1); Salida1.setFont(new Font("consolas", 0, 30)); Salida1.setForeground(new Color(0x000000)); Salida1.setBackground(new Color(0xDDDDDD)); } Icon icono0 = new ImageIcon("C:\Users\Ortuño\Documents\NetBeansProjects\Dialogos\information.png"); JOptionPane.showMessageDialog(null,Salida1,"REGISTRO",JOptionPane.INFORMATION_MESSAGE,icono0); break; case 3: // Abrir el archivo FileInputStream c=new FileInputStream("Registro.txt"); ObjectInputStream cc=new ObjectInputStream(c); obj=(Datos[])cc.readObject(); cc.close(); String nombre=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Nombre a buscar","BUSQUEDA",JOptionPane.PLAIN_MESSAGE); for(int i=0;i<3;i++) if(obj[i].autor.equalsIgnoreCase(nombre)){ JTextArea Salida2= new JTextArea(); String cad2=""; cad2+=("nAUTOR:"+obj[i].autor+" TITULO:"+obj[i].titulo+" PRECIO:"+obj[i].precio+" CODIGO:"+obj[i].codigo+"n"); Salida2.setText(cad2); Salida2.setFont(new Font("consolas", 0, 30)); Salida2.setForeground(new Color(0x000000)); Salida2.setBackground(new Color(0xDDDDDD)); Icon icono1 = new ImageIcon("information.png"); JOptionPane.showMessageDialog(null,Salida2,"RESULTADOS DE BUSQUEDA",JOptionPane.INFORMATION_MESSAGE,icono1); } break; case 4: Icon icono2 = new ImageIcon("information.png"); JOptionPane.showMessageDialog(null,"<html><p style="padding: 10px; font-family: consolas; font-size: 20px;"><b>Hasta luego !","SALIDA",JOptionPane.INFORMATION_MESSAGE,icono2); break; default: Icon icono3 = new ImageIcon("error.png"); JOptionPane.showMessageDialog(null,"<html><p style="padding: 10px; font-family: consolas; font-size: 20px;"><b>Opcion incorrecta !","MENSAJE",JOptionPane.ERROR_MESSAGE,icono3); break; } } while(x==1|x==2|x==3|x!=4); } }
Clase Datos:
package dlibros; //@author Oliver //http://blog2000.blogspot.mx/ import java.io.*; import javax.swing.*; public class Datos implements Serializable{ String autor, titulo, precio, codigo; public void captura(){ autor=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Nombre del autor","AUTOR",JOptionPane.PLAIN_MESSAGE); titulo=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el titulo","TITULO",JOptionPane.PLAIN_MESSAGE); precio=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el precio","PRECIO",JOptionPane.PLAIN_MESSAGE); codigo=JOptionPane.showInputDialog(null,"<html><p style="border-left: solid 20px #0000FF; border-right: solid 20px #0000FF; padding: 10px; font-family: consolas; font-size: 20px;"><b>Introduce el codigo","CODIGO",JOptionPane.PLAIN_MESSAGE); } }
Acerca de mi
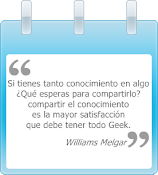
Buscar en el blog
Lista de categorias
- CSS (14)
- Entretenimiento (4)
- HTML (18)
- Iconos (13)
- Inspiración (13)
- JAVA Interfaz (6)
- jQuery (3)
- Lo que NO encontré en Internet (1)
- Programas en C (69)
- Recursos Online (13)
- Trucos (6)
- Widgets (2)